Hi,
I have a UI where the email header is fetched from Message Entity as you can see on the left. What I need is to display the email content when selected. I have gone through the documentation and database table but didn’t find one from where I could display the email body and header data (from email address, to email address, etc.).
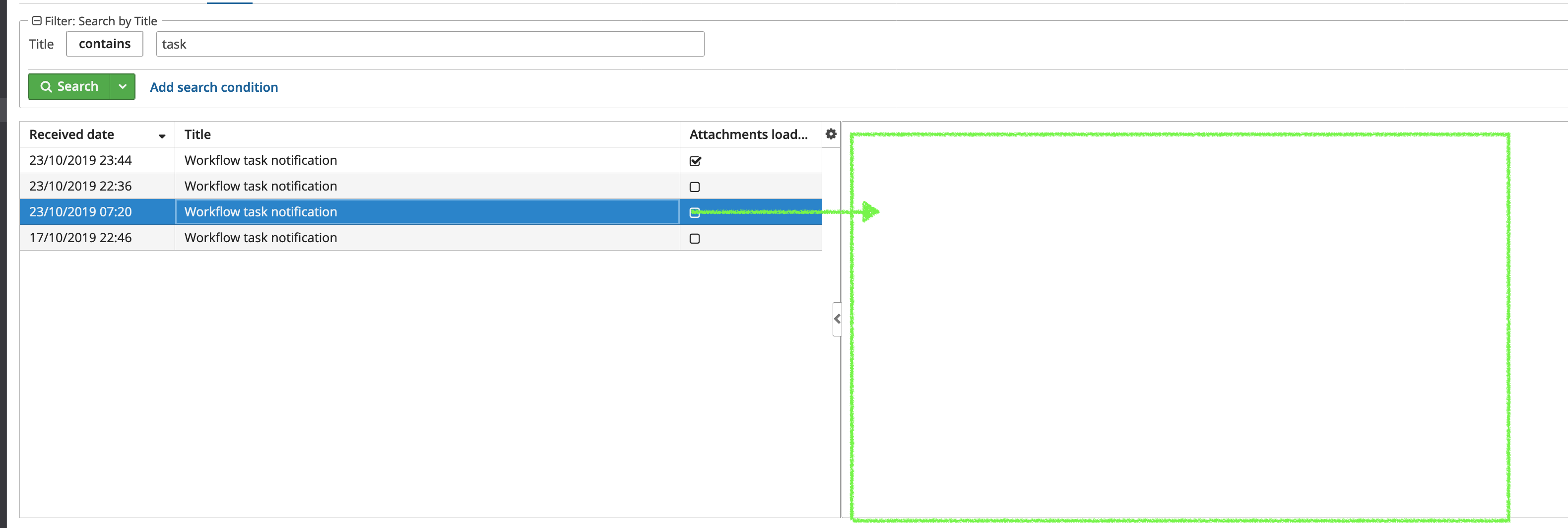
It would be appreciated if someone shares some thoughts.
Hi,
You can use the following code in your application to read message headers:
public void readHeaders(ImapMessage msg) {
ImapMailBox mailBox = msg.getFolder().getMailBox();
String folderName = msg.getFolder().getName();
long uid = msg.getMsgUid();
try {
ImapHelper imapHelper = AppBeans.get("imap_ImapHelper");
IMAPStore store = imapHelper.getStore(mailBox);
try {
IMAPFolder imapFolder = (IMAPFolder) store.getFolder(folderName);
imapFolder.open(Folder.READ_WRITE);
IMAPMessage imapMessage = (IMAPMessage) imapFolder.getMessageByUID(uid);
//Read imap headers
Enumeration<Header> headers = imapMessage.getAllHeaders();
} finally {
store.close();
}
} catch (MessagingException e) {
throw new ImapException(e);
}
}
This code will work on core
application module. It is recommended to read message headers this way in message listener which handle NewEmailImapEvent
.
Regards,
Evgeny
Thanks. Probably I would need a bit more help.
As this is to be called in the middle tier, can you give some hints what I can return to the client layer for from. to, body etc?
I have another scenario, where I have customers who may have multiple email address where I want to display only those emails which are related to the selected customer. Is there any way we can filter the ImapMessge Entity with the email address condition?
List<String> emailIds = null;
int i=0;
String email = getEditedEntity().getProspect().getEmail();
emailIds.add(i, email);
if(getEditedEntity().getCustomerProfile()!=null ) {
i++;
email = getEditedEntity().getCustomerProfile().getEmail();
emailIds.add(i, email);
}
if(getEditedEntity().getProspect()!=null){
//toDO : condition : email addresses of customer and prospect & their contacts.
List<ImapMessage> messageList = dataManager.load(ImapMessage.class)
.query("select e from imap$Message e where (e.emailFrom = :emailIds) OR (e.emailTo = :emailIds) ")
.parameter("emailId", emailIds)
//.view("salesLead-view")
.list();
if(messageList!=null){
}
}